Gender API
Determine gender of first name
https://genderapi.io/api/?name=john
{"name":"john", "q":"john", "gender":"male", "total_names":10855, "probability":100}
Features
Some features of the service we are offering
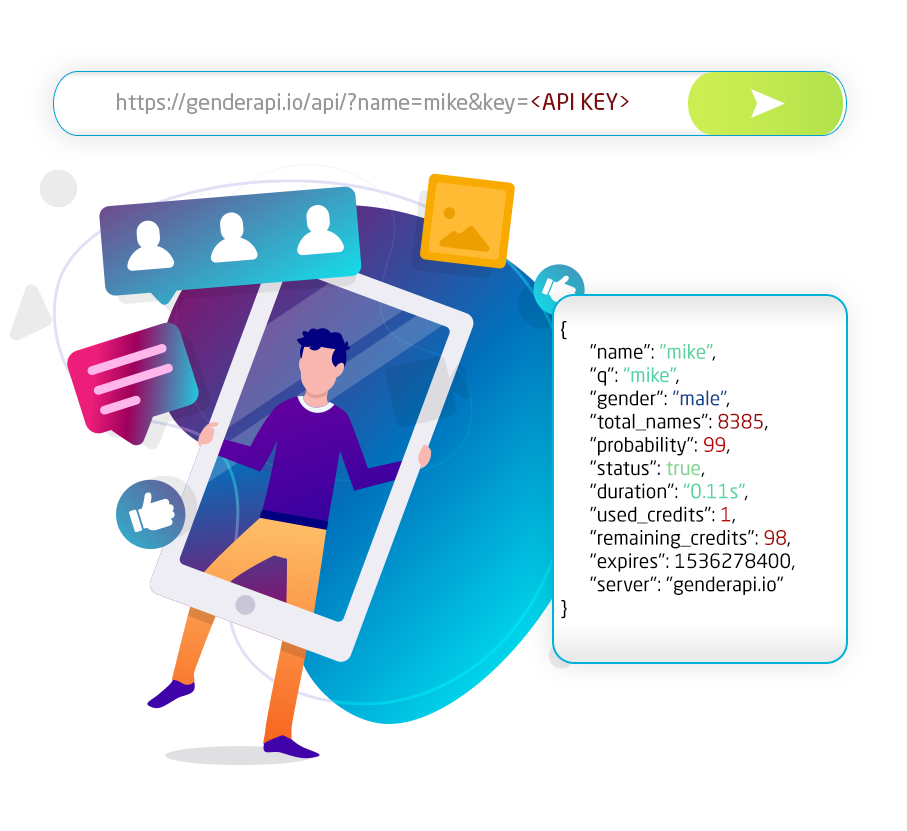
Determine gender by e-mail address
Now we can estimate the gender of the person from the email address. You will love this gorgeous feature :)
Explore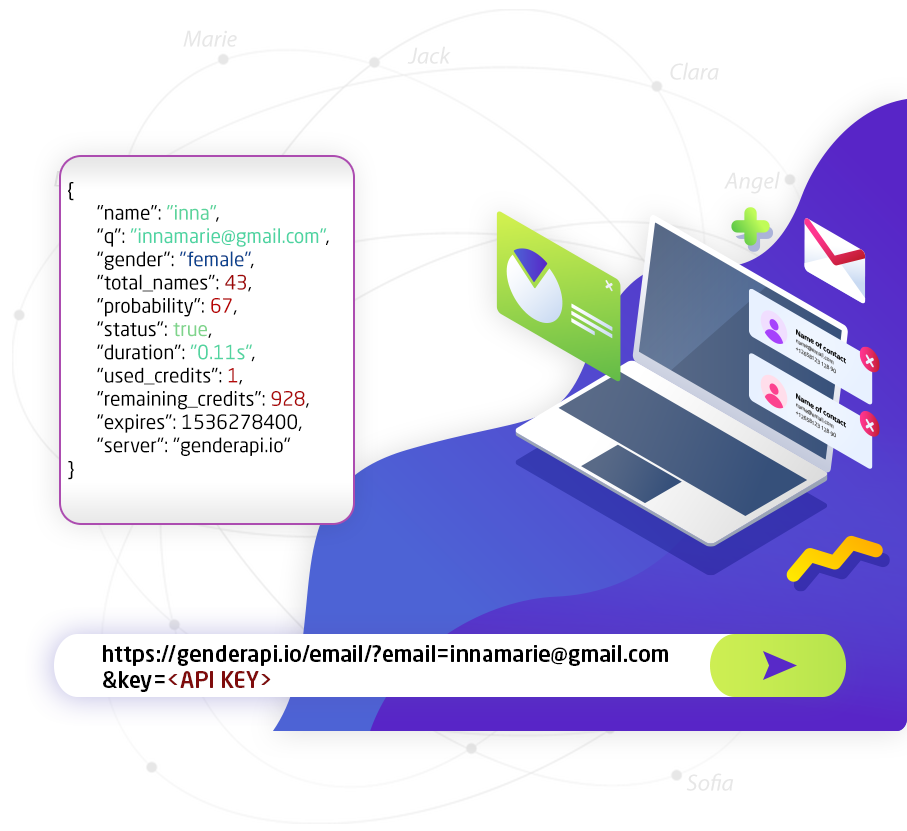
Find Gender From Social Networks
You can query the genders of Facebook, Instagram and Twitter users with their usernames.
Explore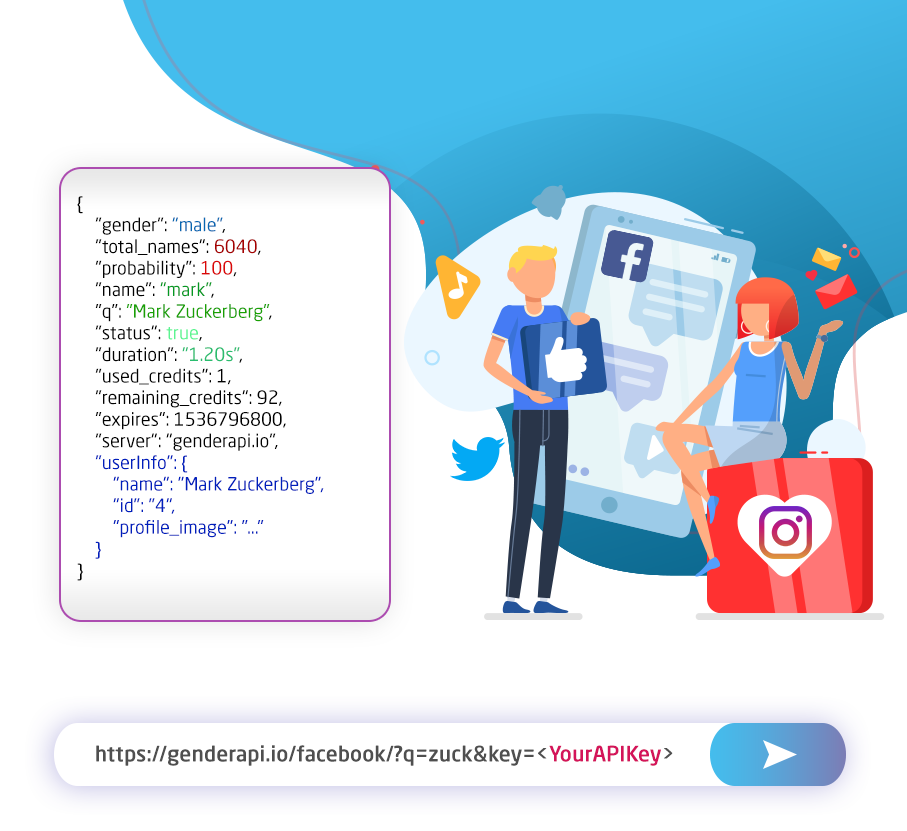
Excel/CSV file upload tool
We thought you might want to analyze your data in a collective way, and we made a great tool for it. All you have to do is select the file you need and your API package, then you can do whatever you want. When your file is finished processing, we will send you an information mail.
Download for MacOS Download for WindowsDaily Live Statistics
We are happy to share our latest statistics with you.
1.13 Requests/Seconds
* These statistics will reset every night. (UTC)
Integrate
Download the integration example we have prepared for the most preferred software languages from our library, and instantly integrate.
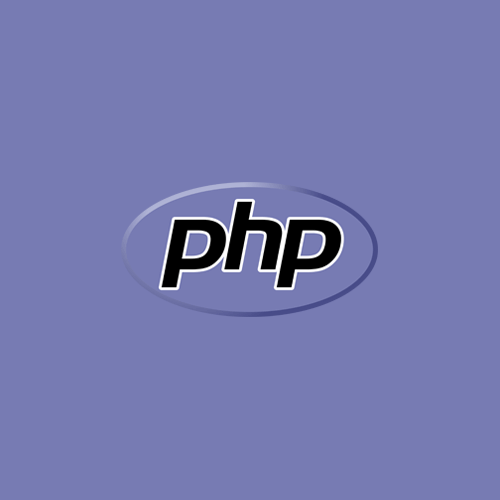

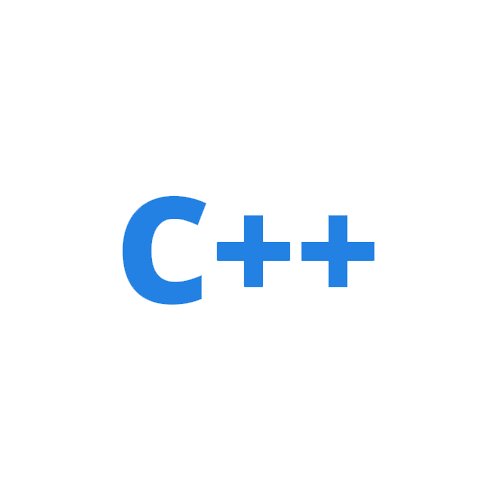





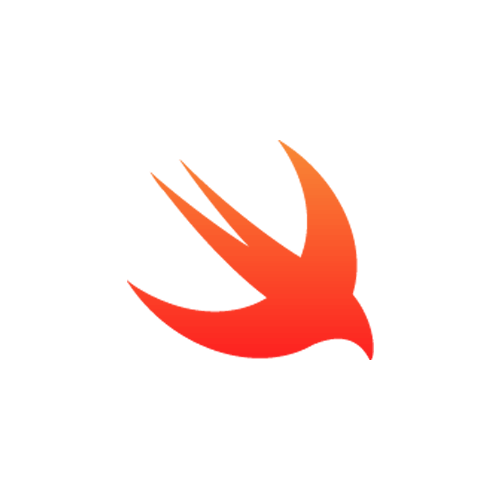
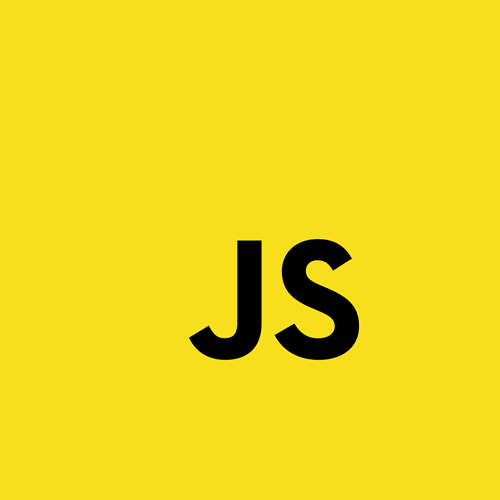
API Documentation
Here are sample codes that we have prepared for you.
<?php
function findGender($name) {
$apiKey = ''; //Your API Key
$getGender = json_decode(file_get_contents('https://genderapi.io/api/?key=' . $apiKey . '&name=' . urlencode($name)));
return $getGender->gender;
}
echo findGender('angela');
Composer command
$ composer require genderapi/php-client
<?php
use GenderApi\GenderApi;
$genderApi = new GenderApi('Your API Key');
$getGender = $genderApi->findGender('britney');
echo $getGender;
// OR, Get Result
$getResult = $genderApi->result('britney');
print_r($getResult);
Python 3.*
import json
from urllib.request import urlopen
apiKey = "" #Your API Key
apiUrl = "https://genderapi.io/api/?name=georgi&key=" + apiKey
result = urlopen(apiUrl).read().decode('utf-8')
getGender = json.loads(result)
print( "Gender: " + getGender["gender"]);
Python 2.*
import json
import urllib2
apiKey = "" #Your API Key
apiUrl = "https://genderapi.io/api/?name=irina&key=" + apiKey
getGender = json.load(urllib2.urlopen(apiUrl))
print "Gender: " + getGender["gender"];
import java.io.IOException;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.net.HttpURLConnection;
import com.google.gson.Gson;
import com.google.gson.JsonObject;
public class Main {
public static void main(String[] args) {
try {
String apiKey = ""; //Your API Key
URL apiUrl = new URL("https://genderapi.io/api/?name=elvan&key=" + apiKey);
HttpURLConnection connect = (HttpURLConnection) apiUrl.openConnection();
if (connect.getResponseCode() != 200) {
throw new RuntimeException("An server error: " + connect.getResponseCode());
}
InputStreamReader iStream = new InputStreamReader(connect.getInputStream());
BufferedReader bReader = new BufferedReader(iStream);
Gson gson = new Gson();
JsonObject jsonOb = gson.fromJson(bReader, JsonObject.class);
String result = jsonOb.get("gender").getAsString();
System.out.println("Gender: " + result); // Gender: male
connect.disconnect();
} catch (IOException error) {
error.printStackTrace();
}
}
}
Javascript & jQuery Example
<!-- Add Javascript & jQuery Library your project -->
<script src="https://genderapi.io/assets/cdn/genderapi.min.js"></script>
//Javascript Code
let API_KEY = ""; //Your API Key
genderApi(API_KEY,"honor",function(result){
console.log(result);
});
//jQuery Code
let API_KEY = ""; //Your API Key
$.genderApi(API_KEY,"honor",function(result){
console.log(result);
});
Javascript Callback Example
<html>
<head>
<meta charset="utf-8">
<title>GenderAPI.io - Javascript Callback Example</title>
</head>
<body>
<input readonly id="result"/>
<script>
function genderApiResult(result) {
var gender = document.getElementById('result');
if (result.gender) {
gender.value = result.gender;
} else {
gender.value = result.errmsg
}
}
</script>
<!-- Add Javascript Callback Library your project -->
<!-- Add your API key -->
<script src="//genderapi.io/api/?name=anna&callback=genderApiResult&key="></script>
</body>